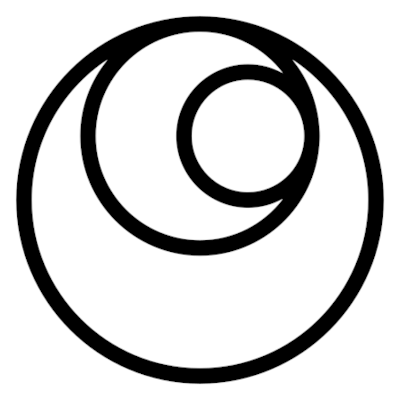
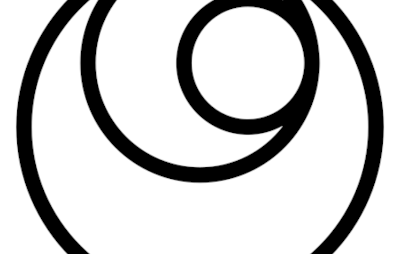
This strategy is based on the popular Fibonacci numbers – a sequence where the next number you get equals the sum of the previous two, starting with 1.
Intro to Fibonacci
Adding the last 2 numbers will give you the next number to use meaning if your last bet was 55 and the bet you just did is 89, then your next bet will be 144. This is what happens when you lose, however there are a few different ways to handle it when you win a bet.
Reset to first bet amount
Bland and plain Fibonacci without any spice (which we’ll see in the 3. and 4.).
Bet Amount | Bet Result | Total Profit |
1 | Loss | -1 |
1 | Loss | -2 |
2 | Loss | -4 |
3 | Loss | -7 |
5 | Win | -2 |
1 | Win | -1 |
1 | Win | 0 |
1 | Loss | -1 |
1 | Loss | -2 |
2 | Loss | -4 |
3 | Loss | -7 |
5 | Loss | -12 |
8 | Loss | -20 |
13 | Loss | -33 |
21 | Loss | -54 |
34 | Win | -20 |
1 | Loss | -21 |
Bet previous bet after win
1.618034 is the number to multiply your loss (rounded) with in most cases to determine the range of the next bet for testing purposes. We know this because if you look in the following table, you’ll be able to notice that according to the Fibonacci sequence, the bet to follow 21 would be 34 seeing as though the bet before 21 was 13 & (21 + 13) = 34. If we use 13 as the dividend and 21 as the divisor we can see the answer is 0.619047619047619. Now if you look closely you’ll see this is very near the number we mentioned above (1.618034) meaning every number before the number you just bet would be needed to recover all the losses of the red streak!
1 | Loss | -1 |
1 | Loss | -2 |
2 | Loss | -4 |
3 | Loss | -7 |
5 | Win | -2 |
3 | Win | 1 |
1 | Win | 2 |
1 | Loss | 1 |
1 | Loss | 0 |
2 | Loss | -2 |
3 | Loss | -5 |
5 | Loss | -10 |
8 | Loss | -18 |
13 | Loss | -31 |
21 | Loss | -52 |
34 | Win | -18 |
21 | Win | 0 |
Recover losses in 2 bets + 1 for profit
Here you can see that we never go down in profit on the 2nd win. The Fibonacci is done the same way as the previous example except that instead of the 2nd bet after the 1st win being the last bet we add enough to this number so that we not only recover the losses but also get 1 unit extra so that our bankroll is constantly increasing in size despite this being a bit more risky than the previous 2 implementations.
1 | Loss | -1 |
1 | Loss | -2 |
2 | Loss | -4 |
3 | Loss | -7 |
5 | Win | -2 |
3 | Win | 1 |
1 | Win | 2 |
1 | Loss | 1 |
1 | Loss | 0 |
2 | Loss | -2 |
3 | Loss | -5 |
5 | Loss | -10 |
8 | Loss | -18 |
13 | Loss | -31 |
21 | Loss | -52 |
34 | Win | -18 |
20 | Loss | 2 |
Developer
If you paste this into your browser, you’ll be able to see the first 15 + 2 Fibonacci numbers. We include the first two which are both the number 1 and simply loop through it 15 times where we calculate the the last 2 numbers in the array by adding them up, and saving the new value at the end. We do this 15 times, however you can easily change it to show how many numbers you want to. Change the 15 in generateFibonacci(15) to whatever number you’d like.
let seed = [1, 1];
function generateFibonacci(num) {
let seed = [1, 1];
for (let i = 0; i < num; i++) {
seed.push(seed[seed.length - 2] + seed[seed.length - 1]);
}
console.log(seed)
}
generateFibonacci(15);
Fibonacci conclusion
This is a unique way to tackle the problem of exponentiation which happens with Martingale. Avoiding exponentiation gives you the chance to survive(bankroll) a longer streak of losses before busting completely. This can be very advantageous on the long run!